錄制視頻顯然要比音頻復雜點,那麼我們一起看看錄制視頻又該如何做呢?
(1)首先,我們肯定要用到攝像頭,因此需要在Manifest文件中聲明使用權限:
<uses-permission android:name="android.permission.CAMERA" />
(2)其次,還要使用一些硬件屬性,那還要做額外的聲明:
<uses-feature android:name="android.hardware.camera"/>
<uses-feature:name="android.hardware.camera.autofocus"/>
<uses-permission android:name="android.permission.RECORD_AUDIO" />
(3)第三,當我們在錄制的時候,通常都想要看到我們正在拍什麼,這就需要預覽,而預覽需要在一個SurfaceView上實現。
一、設計界面
1、首先把record.png、stop.png兩張圖片復制到res/drawable-hdpi文件夾內。
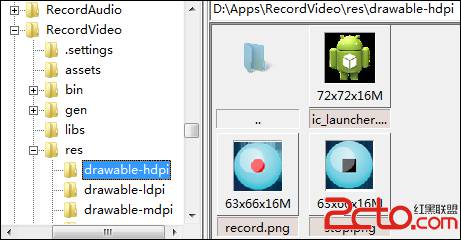
2、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
-
- <ImageButton
- android:id="@+id/record"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/record" />
-
- <ImageButton
- android:id="@+id/stop"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/stop" />
-
- <SurfaceView
- android:id="@+id/surfaceview"
- android:layout_width="300dip"
- android:layout_height="400dip" />
-
-
- </LinearLayout>
二、程序文件
打開“src/com.genwoxue.recordvideo/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.recordvideo;
-
- import java.io.File;
- import java.io.IOException;
- import android.media.MediaRecorder;
- import android.os.Bundle;
- import android.os.Environment;
- import android.view.SurfaceHolder;
- import android.view.SurfaceView;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.ImageButton;
- import android.app.Activity;
-
-
- public class MainActivity extends Activity {
-
- private ImageButton btnRecord=null;
- private ImageButton btnStop=null;
- private SurfaceView mSurfaceView=null;
- private SurfaceHolder mSurfaceHolder=null;
- private MediaRecorder recorder=null;
-
- private File myRecAudioFile=null;
- private File dir=null;
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- mSurfaceView = (SurfaceView) findViewById(R.id.surfaceview);
- mSurfaceHolder = mSurfaceView.getHolder();
- mSurfaceHolder.setKeepScreenOn(true);
- mSurfaceHolder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS);
- btnRecord=(ImageButton)findViewById(R.id.record);
- btnStop=(ImageButton)findViewById(R.id.stop);
-
- File defaultDir = Environment.getExternalStorageDirectory();
- String path = defaultDir.getAbsolutePath()+File.separator+"V"+File.separator;//創建文件夾存放視頻
- dir = new File(path);
- if(!dir.exists()){
- dir.mkdir();
- }
-
- recorder = new MediaRecorder();
-
- //開始錄制視頻
- btnRecord.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- recorder();
- }
- });
-
- //停止錄制視頻
- btnStop.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- recorder.stop();
- recorder.reset();
- recorder.release();
- recorder=null;
- }
- });
-
- }
-
- public void recorder() {
- try {
- myRecAudioFile = File.createTempFile("video", ".3gp",dir);//創建臨時文件
- recorder.setPreviewDisplay(mSurfaceHolder.getSurface());//預覽
- recorder.setVideoSource(MediaRecorder.VideoSource.CAMERA);//視頻源
- recorder.setAudioSource(MediaRecorder.AudioSource.MIC); //錄音源為麥克風
- recorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);//輸出格式為3gp
- recorder.setVideoSize(800, 480);//視頻尺寸
- recorder.setVideoFrameRate(15);//視頻幀頻率
- recorder.setVideoEncoder(MediaRecorder.VideoEncoder.H263);//視頻編碼
- recorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB);//音頻編碼
- recorder.setMaxDuration(10000);//最大期限
- recorder.setOutputFile(myRecAudioFile.getAbsolutePath());//保存路徑
- recorder.prepare();
- recorder.start();
- } catch (IOException e) {
- e.printStackTrace();
- }
- }
-
-
- }