TabActivity
首先Android裡面有個名為TabActivity來給我們方便使用。其中有以下可以關注的函數:
public TabHost getTabHost () 獲得當前TabActivity的TabHost
public TabWidget getTabWidget () 獲得當前TabActivity 的TabWidget
public void setDefaultTab (String tag) 這兩個函數很易懂, 就是設置默認的Tab
public void setDefaultTab (int index) 通過tab名——tag或者index(從0開始)
protected void onRestoreInstanceState (Bundle state) 這 兩個函數的介紹可以
protected void onSaveInstanceState (Bundle outState) 參考 Activity的生命周期
TabHost
那麼我們要用到的Tab載體是TabHost,需要從TabActivity.getTabHost獲取。
現在看看TabHost類,它有3個內嵌類:1個類TabHost.TabSpec,2個接口 TabHost.TabContentFactory和TabHost.OnTabChangeListener。後面會介紹這些類和接口。
TabHost類的一些函數:
public void addTab (TabHost.TabSpec tabSpec) 添加 tab,參數TabHost.TabSpec通過下面的函數返回得到
public TabHost.TabSpec newTabSpec (String tag) 創 建TabHost.TabSpec
public void clearAllTabs () remove所有的Tabs
public int getCurrentTab ()
public String getCurrentTabTag ()
public View getCurrentTabView ()
public View getCurrentView ()
public FrameLayout getTabContentView () 返回Tab content的FrameLayout
public TabWidget getTabWidget ()
public void setCurrentTab (int index) 設置當前的Tab by index
public void setCurrentTabByTag (String tag) 設置當前的Tab by tag
public void setOnTabChangedListener (TabHost.OnTabChangeListener l) 設置TabChanged事件的響應處理
public void setup () 這個函數後面介紹
TabHost.TabSpec
從上面的函數可以知道如何添加tab了,要注意,這裡的Tag(標簽),不是Tab按鈕上的文字。
而要設置tab的label和content,需要設置TabHost.TabSpec類。 引用SDK裡面的話——“A tab has a tab indicator, content, and a tag that is used to keep track of it.”,TabHost.TabSpec就是管理這3個東西:
public String getTag ()
public TabHost.TabSpec setContent
public TabHost.TabSpec setIndicator
我理解這裡的
Indicator 就是Tab上的label,它可以
設置label :
setIndicator (CharSequence label)
或者同時
設置label和icon :
setIndicator (CharSequence label, Drawable icon)
或者直接
指定某個view :
setIndicator (View view)
對於
Content ,就是Tab裡面的內容,可以
設置View的id :
setContent(int viewId)
或者
TabHost.TabContentFactory 的createTabContent(String tag)來處理:
setContent(TabHost.TabContentFactory contentFactory)
或者用
new Intent 來引入其他Activity的內容:
setContent(Intent intent)
主程序代碼
Acitvit裡面的代碼代碼
-
package com.yang.tabletest;
-
-
import android.app.TabActivity;
-
import android.os.Bundle;
-
import android.view.LayoutInflater;
-
import android.widget.TabHost;
-
-
public class TableTestAcitivity extends TabActivity{
-
/** Called when the activity is first created. */
-
@Override
-
public void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
//setContentView(R.layout.main);
-
-
//獲得當前TabActivity的TabHost
-
TabHost tabHost = getTabHost();
-
-
LayoutInflater.from(this).inflate(R.layout.tabs1, tabHost.getTabContentView(), true);
-
-
tabHost.addTab(tabHost.newTabSpec("tab1")
-
.setIndicator("主頁")
-
.setContent(R.id.view1));
-
-
-
tabHost.addTab(tabHost.newTabSpec("tab2")
-
.setIndicator("標題")
-
.setContent(R.id.view2));
-
tabHost.addTab(tabHost.newTabSpec("tab3")
-
.setIndicator("簡介")
-
.setContent(R.id.view3));
-
tabHost.addTab(tabHost.newTabSpec("tab4")
-
.setIndicator("關於")
-
.setContent(R.id.view4));
-
}
-
-
-
}
tabls.xml裡面的代碼
Tabi.xml代碼
-
<frameLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
android:layout_height="fill_parent">
-
-
android:background="@drawable/blue"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent"
-
android:text="@string/tabs_1_tab_1"/>
-
-
android:background="@drawable/red"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent"
-
android:text="@string/tabs_1_tab_2"/>
-
-
android:background="@drawable/green"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent"
-
android:text="@string/tabs_1_tab_3"/>
-
-
android:background="@drawable/green"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent"
-
android:text="@string/tabs_1_tab_4"/>
-
-
</frameLayout>
string.xml的代碼
Java代碼
-
-
-
Hello World, TableTestAcitivity!
-
阿福學習
-
主頁
-
標題
-
關於
-
返回
-
color.xml代碼
Java代碼
-
-
-
#404040ff
-
-
#ff00ff
-
#0ff0ff
-
#c0c0c0ff
-
-
#ffFF33ff
-
#00ffff
-
#808080ff
-
#ff6699ff
-
#66ffffff
-
#000000
-
#FFFFFF
-
第二個例子的Activity代碼
Java代碼
-
package com.yang.tabletest;
-
-
import android.app.TabActivity;
-
import android.os.Bundle;
-
import android.view.View;
-
import android.widget.TabHost;
-
import android.widget.TextView;
-
-
public class TableTestAcitivity extends TabActivity implements TabHost.TabContentFactory{
-
/** Called when the activity is first created. */
-
@Override
-
public void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
-
final TabHost tabHost = getTabHost();
-
tabHost.addTab(tabHost.newTabSpec("tab1")
-
.setIndicator("主頁", getResources().getDrawable(R.drawable.test))
-
.setContent(this));
-
tabHost.addTab(tabHost.newTabSpec("tab2")
-
.setIndicator("標題",getResources().getDrawable(R.drawable.test))
-
.setContent(this));
-
tabHost.addTab(tabHost.newTabSpec("tab3")
-
.setIndicator("關於",getResources().getDrawable(R.drawable.test))
-
.setContent(this));
-
}
-
-
@Override
-
public View createTabContent(String arg0) {
-
final TextView tv = new TextView(this);
-
tv.setText("Content for tab with tag " + arg0);
-
-
return tv;
-
}
-
-
-
-
-
}
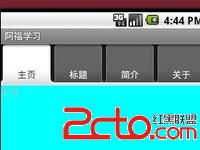
- 大小: 14.3 KB
- TableTest.zip (25.4 KB)
- 下載次數: 563
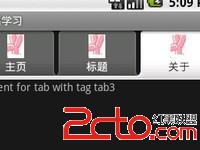
- 大小: 12.6 KB
- TableTest2.zip (29.8 KB)
- 下載次數: 487