單例模式定義:
Ensure a class has only one instance, and provide a global point of access to it.
動態確保某一個類只有一個實例,而且自行實例化並向整個系統提供這個實例。
如上圖所示(截取自《Head First Design Patterns》一書)。
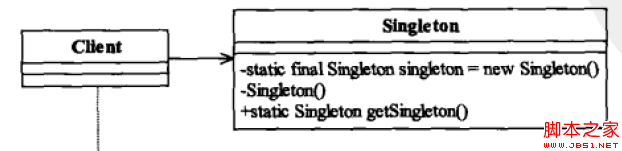
通過使用private的構造函數確保了在一個應用中產生一個實例,並且是自行實例化(在Singleton中自己使用new Singleton())。
具體單例模式有什麼優點呢:
由於單例模式在內存中只有一個實例,減少了內存開銷。
單例模式可以避免對資源的多重占用,例如一個寫文件時,由於只有一個實例存在內存中,避免對同一個資源文件的同時寫操作。
單例模式可以再系統設置全局的訪問點,優化和共享資源訪問。
其中使用到單例模式時,考慮較多的就是多線程的情況下如何防止被多線程同時創建等問題,其中《Head First Design Patterns》使用到“double-checked locking”來降低使用synchronization。
代碼如下:
public class Singleton {
/* The volatile keyword ensures that multiple threads
* handle the uniqueInstance variable correctly when it
* is being initialized to the Singleton instance.
* */
private volatile static Singleton uniqueInstance;
private Singleton() {}
public static Singleton getInstance() {
if(uniqueInstance == null) {
synchronized (Singleton.class) {
if(uniqueInstance == null) {
uniqueInstance = new Singleton();
}
}
}
return uniqueInstance;
}
}
在Android源碼中,使用到單例模式的例子很多,如:
一、 如InputMethodManager類 代碼如下:
public final class InputMethodManager {
static final boolean DEBUG = false;
static final String TAG = "InputMethodManager";
static final Object mInstanceSync = new Object();
static InputMethodManager mInstance;
final IInputMethodManager mService;
final Looper mMainLooper;
創建唯一的實例static InputMethodManager mInstance;
代碼如下:
/**
* Retrieve the global InputMethodManager instance, creating it if it
* doesn't already exist.
* @hide
*/
static public InputMethodManager getInstance(Context context) {
return getInstance(context.getMainLooper());
}
/**
* Internally, the input method manager can't be context-dependent, so
* we have this here for the places that need it.
* @hide
*/
static public InputMethodManager getInstance(Looper mainLooper) {
synchronized (mInstanceSync) {
if (mInstance != null) {
return mInstance;
}
IBinder b = ServiceManager.getService(Context.INPUT_METHOD_SERVICE);
IInputMethodManager service = IInputMethodManager.Stub.asInterface(b);
mInstance = new InputMethodManager(service, mainLooper);
}
return mInstance;
}
防止多線程同時創建實例:
代碼如下:
synchronized (mInstanceSync) {
if (mInstance != null) {
return mInstance;
}
當沒有創建實例對象時,調用mInstance = new InputMethodManager(service, mainLooper);
其中類構造函數如下所示:
代碼如下:
InputMethodManager(IInputMethodManager service, Looper looper) {
mService = service;
mMainLooper = looper;
mH = new H(looper);
mIInputContext = new ControlledInputConnectionWrapper(looper,
mDummyInputConnection);
if (mInstance == null) {
mInstance = this;
}
}
二、BluetoothOppManager類 代碼如下:
public class BluetoothOppManager {
private static final String TAG = "BluetoothOppManager";
private static final boolean V = Constants.VERBOSE;
// 創建private static類實例
private static BluetoothOppManager INSTANCE;
/** Used when obtaining a reference to the singleton instance. */
private static Object INSTANCE_LOCK = new Object();
。。。
/**
* Get singleton instance.
*/
public static BluetoothOppManager getInstance(Context context) {
synchronized (INSTANCE_LOCK) {
if (INSTANCE == null) {
INSTANCE = new BluetoothOppManager();
}
INSTANCE.init(context);
return INSTANCE;
}
}
三、AccessibilityManager類
代碼如下:
public final class AccessibilityManager {
private static final boolean DEBUG = false;
private static final String LOG_TAG = "AccessibilityManager";
/** @hide */
public static final int STATE_FLAG_ACCESSIBILITY_ENABLED = 0x00000001;
/** @hide */
public static final int STATE_FLAG_TOUCH_EXPLORATION_ENABLED = 0x00000002;
static final Object sInstanceSync = new Object();
private static AccessibilityManager sInstance;
...
/**
* Get an AccessibilityManager instance (create one if necessary).
*
* @hide
*/
public static AccessibilityManager getInstance(Context context) {
synchronized (sInstanceSync) {
if (sInstance == null) {
IBinder iBinder = ServiceManager.getService(Context.ACCESSIBILITY_SERVICE);
IAccessibilityManager service = IAccessibilityManager.Stub.asInterface(iBinder);
sInstance = new AccessibilityManager(context, service);
}
}
return sInstance;
}
/**
* Create an instance.
*
* @param context A {@link Context}.
* @param service An interface to the backing service.
*
* @hide
*/
public AccessibilityManager(Context context, IAccessibilityManager service) {
mHandler = new MyHandler(context.getMainLooper());
mService = service;
try {
final int stateFlags = mService.addClient(mClient);
setState(stateFlags);
} catch (RemoteException re) {
Log.e(LOG_TAG, "AccessibilityManagerService is dead", re);
}
}
等等。。。
新年的第一周的開始,從最簡單的單例模式開始記錄自己的學習過程吧~~~