淺談 EventBus,淺談eventbus
概述:
EventBus是一款針對Android優化的發布/訂閱事件總線。
主要功能是替代Intent,Handler,BroadCast在Fragment,Activity,Service。
線程之間傳遞消息.優點是開銷小,代碼更優雅,以及將發送者和接收者解耦。
---------------------------------------------------------------------------------------
下載:
類庫源碼:https://github.com/greenrobot/EventBus
jar包:http://download.csdn.net/detail/yy1300326388/8727699
---------------------------------------------------------------------------------------
使用:
Android Studio 導入第三方類庫太麻煩了,我直接使用了jar包
導入jar包就不用說了吧。
一、EventBus的使用,簡單的來說就是5步:創建一個類(具體使用下面介紹),注冊,發送消息,接收消息,解除注冊
看一個Demo:
實現功能:有兩個Activity,第一個Activity 跳轉第二個Activity,第二個Activity 點擊按鈕發送消息,第一個Activity中的TextView顯示接收到的這個消息信息
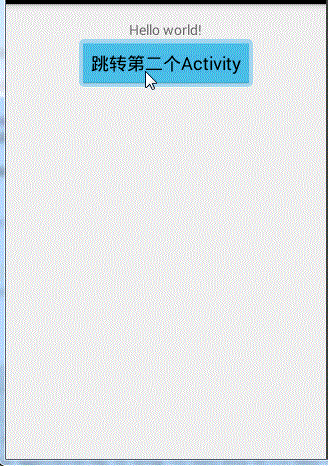
1、寫下兩個Activity的布局

![]()
1 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
2 xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
3 android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
4 android:paddingRight="@dimen/activity_horizontal_margin"
5 android:paddingTop="@dimen/activity_vertical_margin"
6 android:paddingBottom="@dimen/activity_vertical_margin"
7 android:orientation="vertical"
8 tools:context=".MainActivity">
9
10 <TextView
11 android:layout_gravity="center"
12 android:id="@+id/show_msg"
13 android:text="@string/hello_world"
14 android:layout_width="wrap_content"
15 android:layout_height="wrap_content" />
16
17 <Button
18 android:id="@+id/to_second_activity"
19 android:layout_gravity="center"
20 android:layout_width="wrap_content"
21 android:layout_height="wrap_content"
22 android:text="跳轉第二個Activity"/>
23
24 </LinearLayout>
activity_main

![]()
1 <?xml version="1.0" encoding="utf-8"?>
2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
3 android:orientation="vertical" android:layout_width="match_parent"
4 android:layout_height="match_parent">
5
6 <Button
7 android:layout_width="wrap_content"
8 android:layout_height="wrap_content"
9 android:text="發送一個消息"
10 android:id="@+id/send_msg" />
11
12 <Button
13 android:id="@+id/btn_finish"
14 android:text="銷毀這個Activity,返回第一個Activity"
15 android:layout_width="wrap_content"
16 android:layout_height="wrap_content" />
17 </LinearLayout>
activity_second
2、創建一個類,構造方法參數不固定,隨便寫,空類也可以,用於傳遞消息,看具體需求
package com.xqx.com.eventbusdemo;
public class MyMessage {
private String string;
public MyMessage(String string) {
this.string = string;
}
public String getString() {
return string;
}
}
3、在你接收消息的頁面(第一個Activity)注冊和解除注冊EventBus,並且獲取和處理消息
在onCreate()方法中注冊
EventBus.getDefault().register(this);
在onDestroy()方法中取消注冊
EventBus.getDefault().unregister(this);
實現獲取處理消息的方法,這裡先使用onEventMainThread()方法,意思為接收到消息並在UI線程操作
public void onEventMainThread(MyMessage event) {
String msg = "onEventMainThread收到了消息:" + event.getString();
show_msg.setText(msg);
}
完整代碼:

![]()
package com.xqx.com.eventbusdemo;
import android.app.Activity;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import de.greenrobot.event.EventBus;
public class MainActivity extends Activity {
//按鈕,開啟第二個Activity
private Button to_second_activity;
//文本,顯示接收到的消息
private TextView show_msg;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
to_second_activity = (Button) findViewById(R.id.to_second_activity);
show_msg = (TextView) findViewById(R.id.show_msg);
//注冊
EventBus.getDefault().register(this);
//點擊按鈕進入到第二個Activity
to_second_activity.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this,SecondActivity.class));
}
});
}
//接收消息並處理
public void onEventMainThread(MyMessage event) {
String msg = "onEventMainThread收到了消息:" + event.getString();
show_msg.setText(msg);
}
@Override
protected void onDestroy() {
super.onDestroy();
// 解除注冊
EventBus.getDefault().unregister(this);
}
}
MainActivity.class
4、在要發送消息的頁面發送消息
發送消息很簡單,參數是你自己寫的那個類
EventBus.getDefault().post(new MyMessage("this is a message"));
完整代碼:

![]()
package com.xqx.com.eventbusdemo;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import de.greenrobot.event.EventBus;
public class SecondActivity extends Activity{
private Button send_msg;
private Button btn_finish;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
send_msg = (Button) findViewById(R.id.send_msg);
btn_finish = (Button) findViewById(R.id.btn_finish);
send_msg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EventBus.getDefault().post(new MyMessage("this is a message"));
}
});
btn_finish.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
SecondActivity.class
---------------------------------------------------------------------------------------
EventBus其他知識說明:
1、EventBus有四個不同的消息接收處理方法:
onEvent:
使用onEvent,那麼該事件在哪個線程發布出來的,onEvent就會在這個線程中運行,也就是說發布事件和接收事件線程在同一個線程。使用這個方法時,在onEvent方法中不能執行耗時操作,如果執行耗時操作容易導致事件分發延遲。
onEventMainThread:
使用onEventMainThread,那麼不論事件是在哪個線程中發布出來的,onEventMainThread都會在UI線程中執行,接收事件就會在UI線程中運行,這個在Android中是非常有用的,因為在Android中只能在UI線程中跟新UI,所以在onEvnetMainThread方法中是不能執行耗時操作的。
onEventBackground:
使用onEventBackgrond,那麼如果事件是在UI線程中發布出來的,那麼onEventBackground就會在子線程中運行,如果事件本來就是子線程中發布出來的,那麼onEventBackground函數直接在該子線程中執行。
onEventAsync:
使用onEventAsync,那麼無論事件在哪個線程發布,都會創建新的子線程在執行onEventAsync.
2、如果有多個地方發送消息,並且有多個消息處理函數,怎麼確定哪個消息處理方法處理哪些消息呢?
這就看四個消息處理方法的參數。發送消息的參數是某一個類,接收的也必須是這個類,否則接收不到。如有有多個OnEvent()方法參數相同,那麼這些方法都可以接收到消息。
---------------------------------------------------------------------------------------
總結:
register(注冊)會把當前類中匹配的方法(onEvent開頭的),存入一個map,而post會根據實參去map查找進行反射調用