自定義控件之 圓形 / 圓角 ImageView,圓角imageview
一、問題在哪裡?
問題來源於app開發中一個很常見的場景——用戶頭像要展示成圓的:
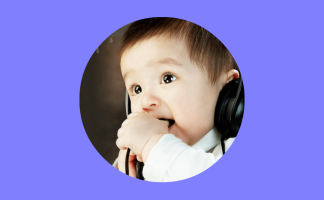
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
二、怎麼搞?
機智的我,第一想法就是,切一張中間圓形透明、四周與底色相同、尺寸與頭像相同的蒙板圖片,蓋在頭像上不就完事了嘛,哈哈哈!
在背景純色的前提下,這的確能簡單解決問題,但是如果背景沒有這麼簡單呢?
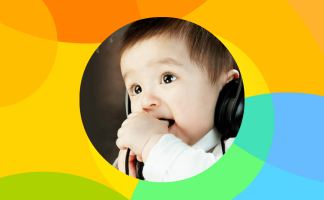
在這種不規則背景下,有兩個問題:
1) 背景圖常常是適應手機寬度縮放,而頭像的尺寸又是固定寬高DP的,所以固定的蒙板圖片是沒法保證在不同機型上都和背景圖案吻合的。
2) 在這種非純色背景下,哪天想調整一下頭像位置就得重新換圖片蒙板,實在是太難維護了……
所以呢,既然頭像圖片肯定是方的,那就就讓ImageView圓起來吧。
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
三、開始干活
基本思路是,自定義一個ImageView,通過重寫onDraw方法畫出一個圓形的圖片來:
1 public class ImageViewPlus extends ImageView{
2 private Paint mPaintBitmap = new Paint(Paint.ANTI_ALIAS_FLAG);
3 private Bitmap mRawBitmap;
4 private BitmapShader mShader;
5 private Matrix mMatrix = new Matrix();
6
7 public ImageViewPlus(Context context, AttributeSet attrs) {
8 super(context, attrs);
9 }
10
11 @Override
12 protected void onDraw(Canvas canvas) {
13 Bitmap rawBitmap = getBitmap(getDrawable());
14 if (rawBitmap != null){
15 int viewWidth = getWidth();
16 int viewHeight = getHeight();
17 int viewMinSize = Math.min(viewWidth, viewHeight);
18 float dstWidth = viewMinSize;
19 float dstHeight = viewMinSize;
20 if (mShader == null || !rawBitmap.equals(mRawBitmap)){
21 mRawBitmap = rawBitmap;
22 mShader = new BitmapShader(mRawBitmap, TileMode.CLAMP, TileMode.CLAMP);
23 }
24 if (mShader != null){
25 mMatrix.setScale(dstWidth / rawBitmap.getWidth(), dstHeight / rawBitmap.getHeight());
26 mShader.setLocalMatrix(mMatrix);
27 }
28 mPaintBitmap.setShader(mShader);
29 float radius = viewMinSize / 2.0f;
30 canvas.drawCircle(radius, radius, radius, mPaintBitmap);
31 } else {
32 super.onDraw(canvas);
33 }
34 }
35
36 private Bitmap getBitmap(Drawable drawable){
37 if (drawable instanceof BitmapDrawable){
38 return ((BitmapDrawable)drawable).getBitmap();
39 } else if (drawable instanceof ColorDrawable){
40 Rect rect = drawable.getBounds();
41 int width = rect.right - rect.left;
42 int height = rect.bottom - rect.top;
43 int color = ((ColorDrawable)drawable).getColor();
44 Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
45 Canvas canvas = new Canvas(bitmap);
46 canvas.drawARGB(Color.alpha(color), Color.red(color), Color.green(color), Color.blue(color));
47 return bitmap;
48 } else {
49 return null;
50 }
51 }
52 }
分析一下代碼:
canvas.drawCircle 決定了畫出來的形狀是圓形,而圓形的內容則是通過 mPaintBitmap.setShader 搞定的。
其中,BitmapShader需要設置Bitmap填充ImageView的方式(CLAMP:拉伸邊緣, MIRROR:鏡像, REPEAT:整圖重復)。
這裡其實設成什麼不重要,因為我們實際需要的是將Bitmap按比例縮放成跟ImageView一樣大,而不是預置的三種效果。
所以,別忘了 mMatrix.setScale 和 mShader.setLocalMatrix 一起用,將圖片縮放一下。
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
四、更多玩法 —— 支持邊框
看下面的效果圖,如果想給圓形的頭像上加一個邊框,該怎麼搞呢?

![]()
1 public class ImageViewPlus extends ImageView{
2 private Paint mPaintBitmap = new Paint(Paint.ANTI_ALIAS_FLAG);
3 private Paint mPaintBorder = new Paint(Paint.ANTI_ALIAS_FLAG);
4 private Bitmap mRawBitmap;
5 private BitmapShader mShader;
6 private Matrix mMatrix = new Matrix();
7 private float mBorderWidth = dip2px(15);
8 private int mBorderColor = 0xFF0080FF;
9
10 public ImageViewPlus(Context context, AttributeSet attrs) {
11 super(context, attrs);
12 }
13
14 @Override
15 protected void onDraw(Canvas canvas) {
16 Bitmap rawBitmap = getBitmap(getDrawable());
17 if (rawBitmap != null){
18 int viewWidth = getWidth();
19 int viewHeight = getHeight();
20 int viewMinSize = Math.min(viewWidth, viewHeight);
21 float dstWidth = viewMinSize;
22 float dstHeight = viewMinSize;
23 if (mShader == null || !rawBitmap.equals(mRawBitmap)){
24 mRawBitmap = rawBitmap;
25 mShader = new BitmapShader(mRawBitmap, TileMode.CLAMP, TileMode.CLAMP);
26 }
27 if (mShader != null){
28 mMatrix.setScale((dstWidth - mBorderWidth * 2) / rawBitmap.getWidth(), (dstHeight - mBorderWidth * 2) / rawBitmap.getHeight());
29 mShader.setLocalMatrix(mMatrix);
30 }
31 mPaintBitmap.setShader(mShader);
32 mPaintBorder.setStyle(Paint.Style.STROKE);
33 mPaintBorder.setStrokeWidth(mBorderWidth);
34 mPaintBorder.setColor(mBorderColor);
35 float radius = viewMinSize / 2.0f;
36 canvas.drawCircle(radius, radius, radius - mBorderWidth / 2.0f, mPaintBorder);
37 canvas.translate(mBorderWidth, mBorderWidth);
38 canvas.drawCircle(radius - mBorderWidth, radius - mBorderWidth, radius - mBorderWidth, mPaintBitmap);
39 } else {
40 super.onDraw(canvas);
41 }
42 }
43
44 private Bitmap getBitmap(Drawable drawable){
45 if (drawable instanceof BitmapDrawable){
46 return ((BitmapDrawable)drawable).getBitmap();
47 } else if (drawable instanceof ColorDrawable){
48 Rect rect = drawable.getBounds();
49 int width = rect.right - rect.left;
50 int height = rect.bottom - rect.top;
51 int color = ((ColorDrawable)drawable).getColor();
52 Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
53 Canvas canvas = new Canvas(bitmap);
54 canvas.drawARGB(Color.alpha(color), Color.red(color), Color.green(color), Color.blue(color));
55 return bitmap;
56 } else {
57 return null;
58 }
59 }
60
61 private int dip2px(int dipVal)
62 {
63 float scale = getResources().getDisplayMetrics().density;
64 return (int)(dipVal * scale + 0.5f);
65 }
66 }
View Code
看代碼中,加邊框實際上就是用實心純色的 Paint 畫了一個圓邊,在此基礎上畫上原來的頭像即可。
需要的注意的地方有三個:
1) 圓框的半徑不是 radius ,而應該是 radius - mBorderWidth / 2.0f 。想象著拿著筆去畫線,線其實是畫在右圖中白色圈的位置,只不過它很粗。
2) 在ImageView大小不變的基礎上,頭像的實際大小要比沒有邊框的時候小了,所以 mMatrix.setScale 的時候要把邊框的寬度去掉。
3) 畫頭像Bitmap的時候不能直接 canvas.drawCircle(radius, radius, radius - mBorderWidth, mPaintBitmap) ,這樣你會發現頭像的右側和下方邊緣被拉伸了(右圖)
為什麼呢?因為 Paint 默認是以左上角為基准開始繪制的,此時頭像的實際區域是右圖中的紅框,而超過紅框的部分(圓形的右側和下方),自然被 TileMode.CLAMP效果沿邊緣拉伸了。
所以,需要通過挪動坐標系的位置和調整圓心,才能把頭像畫在正確的區域(右圖綠框)中。
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
五、更多玩法 —— 支持xml配置
既然有了邊框,那如果想配置邊框的寬度和顏色該如何是好呢?
基本上兩個思路:
1) 給ImageViewPlus加上set接口,設置完成之後通過 invalidate(); 重繪一下即可;
2) 在xml裡就支持配置一些自定義屬性,這樣用起來會方便很多。
這裡重點說一下支持xml配置自定義屬性。
自定義控件要支持xml配置自定義屬性的話,首先需要在 \res\values 裡去定義屬性:

![]()
1 <?xml version="1.0" encoding="utf-8"?>
2 <resources>
3 <attr name="borderColor" format="color" />
4 <attr name="borderWidth" format="dimension" />
5
6 <declare-styleable name="ImageViewPlus">
7 <attr name="borderColor" />
8 <attr name="borderWidth" />
9 </declare-styleable>
10 </resources>
View attrs_imageviewplus.xml
然後在ImageViewPlus的構造函數中去讀取這些自定義屬性:

![]()
1 private static final int DEFAULT_BORDER_COLOR = Color.TRANSPARENT;
2 private static final int DEFAULT_BORDER_WIDTH = 0;
3
4 public ImageViewPlus(Context context, AttributeSet attrs) {
5 super(context, attrs);
6 //取xml文件中設定的參數
7 TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.ImageViewPlus);
8 mBorderColor = ta.getColor(R.styleable.ImageViewPlus_borderColor, DEFAULT_BORDER_COLOR);
9 mBorderWidth = ta.getDimensionPixelSize(R.styleable.ImageViewPlus_borderWidth, dip2px(DEFAULT_BORDER_WIDTH));
10 ta.recycle();
11 }
View Code
在xml布局中使用自定義屬性:

![]()
1 <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
2 xmlns:tools="http://schemas.android.com/tools"
3 xmlns:snser="http://schemas.android.com/apk/res/cc.snser.imageviewplus"
4 android:layout_width="match_parent"
5 android:layout_height="match_parent"
6 android:background="@drawable/wallpaper"
7 android:orientation="vertical"
8 tools:context="${relativePackage}.${activityClass}" >
9
10 <cc.snser.imageviewplus.ImageViewPlus
11 android:id="@+id/imgplus"
12 android:layout_width="200dp"
13 android:layout_height="300dp"
14 android:layout_marginBottom="50dp"
15 android:layout_centerHorizontal="true"
16 android:layout_alignParentBottom="true"
17 android:src="@drawable/img_square"
18 snser:borderColor="#FF0080FF"
19 snser:borderWidth="15dp" />
20
21 </RelativeLayout>
View Code
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
六、更多玩法 —— 圓角ImageView
搞定了圓形ImageView以及對應的邊框,那如何實現下面這種圓角的ImageView呢?
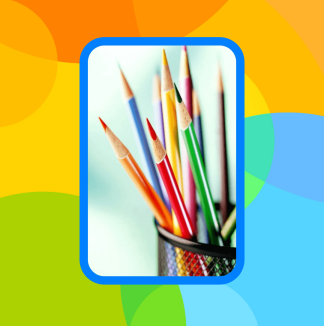
其實原理上一樣,把 canvas.drawCircle 對應改成 canvas.drawRoundRect 就OK了,直接貼代碼吧:

![]()
1 public class ImageViewPlus extends ImageView{
2 /**
3 * android.widget.ImageView
4 */
5 public static final int TYPE_NONE = 0;
6 /**
7 * 圓形
8 */
9 public static final int TYPE_CIRCLE = 1;
10 /**
11 * 圓角矩形
12 */
13 public static final int TYPE_ROUNDED_RECT = 2;
14
15 private static final int DEFAULT_TYPE = TYPE_NONE;
16 private static final int DEFAULT_BORDER_COLOR = Color.TRANSPARENT;
17 private static final int DEFAULT_BORDER_WIDTH = 0;
18 private static final int DEFAULT_RECT_ROUND_RADIUS = 0;
19
20 private int mType;
21 private int mBorderColor;
22 private int mBorderWidth;
23 private int mRectRoundRadius;
24
25 private Paint mPaintBitmap = new Paint(Paint.ANTI_ALIAS_FLAG);
26 private Paint mPaintBorder = new Paint(Paint.ANTI_ALIAS_FLAG);
27
28 private RectF mRectBorder = new RectF();
29 private RectF mRectBitmap = new RectF();
30
31 private Bitmap mRawBitmap;
32 private BitmapShader mShader;
33 private Matrix mMatrix = new Matrix();
34
35 public ImageViewPlus(Context context, AttributeSet attrs) {
36 super(context, attrs);
37 //取xml文件中設定的參數
38 TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.ImageViewPlus);
39 mType = ta.getInt(R.styleable.ImageViewPlus_type, DEFAULT_TYPE);
40 mBorderColor = ta.getColor(R.styleable.ImageViewPlus_borderColor, DEFAULT_BORDER_COLOR);
41 mBorderWidth = ta.getDimensionPixelSize(R.styleable.ImageViewPlus_borderWidth, dip2px(DEFAULT_BORDER_WIDTH));
42 mRectRoundRadius = ta.getDimensionPixelSize(R.styleable.ImageViewPlus_rectRoundRadius, dip2px(DEFAULT_RECT_ROUND_RADIUS));
43 ta.recycle();
44 }
45
46 @Override
47 protected void onDraw(Canvas canvas) {
48 Bitmap rawBitmap = getBitmap(getDrawable());
49
50 if (rawBitmap != null && mType != TYPE_NONE){
51 int viewWidth = getWidth();
52 int viewHeight = getHeight();
53 int viewMinSize = Math.min(viewWidth, viewHeight);
54 float dstWidth = mType == TYPE_CIRCLE ? viewMinSize : viewWidth;
55 float dstHeight = mType == TYPE_CIRCLE ? viewMinSize : viewHeight;
56 float halfBorderWidth = mBorderWidth / 2.0f;
57 float doubleBorderWidth = mBorderWidth * 2;
58
59 if (mShader == null || !rawBitmap.equals(mRawBitmap)){
60 mRawBitmap = rawBitmap;
61 mShader = new BitmapShader(mRawBitmap, TileMode.CLAMP, TileMode.CLAMP);
62 }
63 if (mShader != null){
64 mMatrix.setScale((dstWidth - doubleBorderWidth) / rawBitmap.getWidth(), (dstHeight - doubleBorderWidth) / rawBitmap.getHeight());
65 mShader.setLocalMatrix(mMatrix);
66 }
67
68 mPaintBitmap.setShader(mShader);
69 mPaintBorder.setStyle(Paint.Style.STROKE);
70 mPaintBorder.setStrokeWidth(mBorderWidth);
71 mPaintBorder.setColor(mBorderWidth > 0 ? mBorderColor : Color.TRANSPARENT);
72
73 if (mType == TYPE_CIRCLE){
74 float radius = viewMinSize / 2.0f;
75 canvas.drawCircle(radius, radius, radius - halfBorderWidth, mPaintBorder);
76 canvas.translate(mBorderWidth, mBorderWidth);
77 canvas.drawCircle(radius - mBorderWidth, radius - mBorderWidth, radius - mBorderWidth, mPaintBitmap);
78 } else if (mType == TYPE_ROUNDED_RECT){
79 mRectBorder.set(halfBorderWidth, halfBorderWidth, dstWidth - halfBorderWidth, dstHeight - halfBorderWidth);
80 mRectBitmap.set(0.0f, 0.0f, dstWidth - doubleBorderWidth, dstHeight - doubleBorderWidth);
81 float borderRadius = mRectRoundRadius - halfBorderWidth > 0.0f ? mRectRoundRadius - halfBorderWidth : 0.0f;
82 float bitmapRadius = mRectRoundRadius - mBorderWidth > 0.0f ? mRectRoundRadius - mBorderWidth : 0.0f;
83 canvas.drawRoundRect(mRectBorder, borderRadius, borderRadius, mPaintBorder);
84 canvas.translate(mBorderWidth, mBorderWidth);
85 canvas.drawRoundRect(mRectBitmap, bitmapRadius, bitmapRadius, mPaintBitmap);
86 }
87 } else {
88 super.onDraw(canvas);
89 }
90 }
91
92 private int dip2px(int dipVal)
93 {
94 float scale = getResources().getDisplayMetrics().density;
95 return (int)(dipVal * scale + 0.5f);
96 }
97
98 private Bitmap getBitmap(Drawable drawable){
99 if (drawable instanceof BitmapDrawable){
100 return ((BitmapDrawable)drawable).getBitmap();
101 } else if (drawable instanceof ColorDrawable){
102 Rect rect = drawable.getBounds();
103 int width = rect.right - rect.left;
104 int height = rect.bottom - rect.top;
105 int color = ((ColorDrawable)drawable).getColor();
106 Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
107 Canvas canvas = new Canvas(bitmap);
108 canvas.drawARGB(Color.alpha(color), Color.red(color), Color.green(color), Color.blue(color));
109 return bitmap;
110 } else {
111 return null;
112 }
113 }
114 }
View ImageViewPlus.java

![]()
1 <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
2 xmlns:tools="http://schemas.android.com/tools"
3 xmlns:snser="http://schemas.android.com/apk/res/cc.snser.imageviewplus"
4 android:layout_width="match_parent"
5 android:layout_height="match_parent"
6 android:background="@drawable/wallpaper"
7 android:orientation="vertical"
8 tools:context="${relativePackage}.${activityClass}" >
9
10 <cc.snser.imageviewplus.ImageViewPlus
11 android:id="@+id/imgplus"
12 android:layout_width="200dp"
13 android:layout_height="300dp"
14 android:layout_marginBottom="50dp"
15 android:layout_centerHorizontal="true"
16 android:layout_alignParentBottom="true"
17 android:src="@drawable/img_rectangle"
18 snser:type="rounded_rect"
19 snser:borderColor="#FF0080FF"
20 snser:borderWidth="10dp"
21 snser:rectRoundRadius="30dp" />
22
23 </RelativeLayout>
View layout

![]()
1 <?xml version="1.0" encoding="utf-8"?>
2 <resources>
3 <attr name="type">
4 <enum name="none" value="0" />
5 <enum name="circle" value="1" />
6 <enum name="rounded_rect" value="2" />
7 </attr>
8 <attr name="borderColor" format="color" />
9 <attr name="borderWidth" format="dimension" />
10 <attr name="rectRoundRadius" format="dimension" />
11
12 <declare-styleable name="ImageViewPlus">
13 <attr name="type" />
14 <attr name="borderColor" />
15 <attr name="borderWidth" />
16 <attr name="rectRoundRadius" />
17 </declare-styleable>
18 </resources>
View attrs_imageviewplus.xml
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]
七、完結
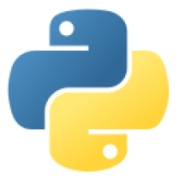
[轉載請保留本文地址:http://www.cnblogs.com/goagent/p/5159126.html]